More pros and cons of architecture selection from IBM.
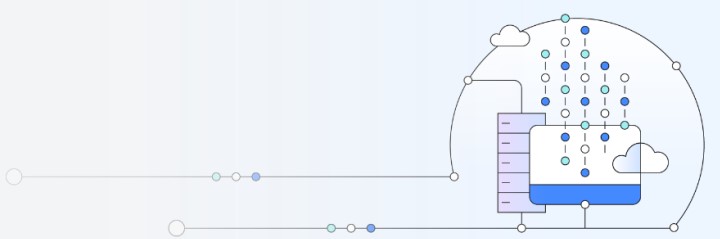
More pros and cons of architecture selection from IBM.
When MVC became the latest fad for web development, I immediately liked it. The clearly defined design pattern made natural sense to me. It is very nice setup for smaller projects. However, as my corporate-level applications continue to grow I began to fumble with scalability and testing. There is no one-size-fits all and the initial magic has to come from architectural decisions for projects on the whole.
The ideal design pattern for your project depends on its specific requirements. Consider factors like project size, complexity, desired level of separation of concerns, and development team experience. Below is a summary of the spawns of MVC as simply defined by the folks at Orient Software :
The Model-View-Presenter (MVP) pattern introduces a Presenter component as an intermediary between the Model and the View. Unlike MVC, the View in MVP has no direct knowledge of the Model. Instead, the Presenter fetches data from the Model and prepares it for the View in a suitable format. The View then displays the data and sends user interactions back to the Presenter. This separation promotes an even stricter separation of concerns compared to MVC.
Advantages and Use Cases:
MVP is a good choice for projects that require a clear separation of UI logic and business logic, particularly for complex user interfaces. It’s also well-suited for scenarios where testability and maintainability are critical aspects of the development process.
The Model-View-ViewModel (MVVM) pattern introduces a ViewModel, which acts as a data intermediary between the Model and the View. The ViewModel retrieves data from the Model, prepares it for presentation, and exposes it to the View through data binding mechanisms. User interactions within the View are then channeled through the ViewModel, which can update the Model and notify the View of any changes.
Benefits and Scenarios:
MVVM is a popular choice for building complex and dynamic user interfaces, particularly in desktop and mobile app development frameworks that support data binding. It’s also advantageous for projects where frequent UI updates and data synchronization are important.
The Model-View-Intent (MVI) architecture pattern is a relatively new approach that uses unidirectional data flow. In MVI, the View dispatches user intents (actions) to a central store. This store holds the application state and updates the Model based on the received intents. The store then emits a new state back to the View, triggering a re-render with the updated data.
Advantages and Considerations:
MVI is particularly well-suited for building reactive applications where state management and immutability are key concerns. However, its adoption might require developers to be familiar with functional programming concepts.
For instance, if testability and clear separation of UI logic from business logic are priorities, MVP might be a strong contender. If you’re building a data-driven application with frequent UI updates, MVVM’s two-way data binding could be a significant benefit. And for highly reactive applications where state management is crucial, MVI could be a compelling choice.
Simply put, the Singleton pattern ensures that only one instance of the class exists and typically provides a well-known, i.e., global point for accessing it. The Factory pattern defines an interface for creating objects (no limitation on how many) and usually abstracts the control of which class to instantiate.